陣列的操作
filter()、map()、reduce()、sort()
目標 / 題目
使用 array.prototype 依據不同需求條件使用不同的 method 篩選出正確的資料。
題目 1~5. 對inventors資料做篩選。
1
2
3
4
5
6
7
8
9
10
11
12
13
14const inventors = [
{ first: 'Albert', last: 'Einstein', year: 1879, passed: 1955 },
{ first: 'Isaac', last: 'Newton', year: 1643, passed: 1727 },
{ first: 'Galileo', last: 'Galilei', year: 1564, passed: 1642 },
{ first: 'Marie', last: 'Curie', year: 1867, passed: 1934 },
{ first: 'Johannes', last: 'Kepler', year: 1571, passed: 1630 },
{ first: 'Nicolaus', last: 'Copernicus', year: 1473, passed: 1543 },
{ first: 'Max', last: 'Planck', year: 1858, passed: 1947 },
{ first: 'Katherine', last: 'Blodgett', year: 1898, passed: 1979 },
{ first: 'Ada', last: 'Lovelace', year: 1815, passed: 1852 },
{ first: 'Sarah E.', last: 'Goode', year: 1855, passed: 1905 },
{ first: 'Lise', last: 'Meitner', year: 1878, passed: 1968 },
{ first: 'Hanna', last: 'Hammarström', year: 1829, passed: 1909 },
];題目 6. 列出網址中所有包含’de’的路名。
網址:連結題目 7. 依據lastName排序所有people的資料。
1
const people = ['Beck, Glenn', 'Becker, Carl', 'Beckett, Samuel', 'Beddoes, Mick', 'Beecher, Henry', 'Beethoven, Ludwig', 'Begin, Menachem', 'Belloc, Hilaire', 'Bellow, Saul', 'Benchley, Robert', 'Benenson, Peter', 'Ben-Gurion, David', 'Benjamin, Walter', 'Benn, Tony', 'Bennington, Chester', 'Benson, Leana', 'Bent, Silas', 'Bentsen, Lloyd', 'Berger, Ric', 'Bergman, Ingmar', 'Berio, Luciano', 'Berle, Milton', 'Berlin, Irving', 'Berne, Eric', 'Bernhard, Sandra', 'Berra, Yogi', 'Berry, Halle', 'Berry, Wendell', 'Bethea, Erin', 'Bevan, Aneurin', 'Bevel, Ken', 'Biden, Joseph', 'Bierce, Ambrose', 'Biko, Steve', 'Billings, Josh', 'Biondo, Frank', 'Birrell, Augustine', 'Black, Elk', 'Blair, Robert', 'Blair, Tony', 'Blake, William'];
題目 8. 列出data內每個種類的數量。
1
const data = ['car', 'car', 'truck', 'truck', 'bike', 'walk', 'car', 'van', 'bike', 'walk', 'car', 'van', 'car', 'truck' ];
實作要點
- 利用 filter(),過濾出 1500 年代出生的人。
- 利用 map(),得到一個陣列(inventors’ first and last names)。
- 利用 sort(),將陣列的「birthdate」資料由大至小排序(inventors’s birthdate, oldest to youngest)
- 利用 reduce(),加總所有人的年齡。
- 利用 sort(),將陣列資料依年齡大小作排序。
- 利用 filter(),列出 wiki 資料中所有包含’de’的路名
- 利用 sort(),將 people 的資料依 lastName 做排序。
- 利用 reduce(),計算 data 內每個種類的數量。
實作
1. 過濾出 1500 年代出生的人
→ filter()
1 | const ans1 = inventors.filter(function(inventor) { |

2. 將 inventors 的 first 和 last 組成陣列
→ map()
1 | const ans2 = inventors.map(function(inventor) { |
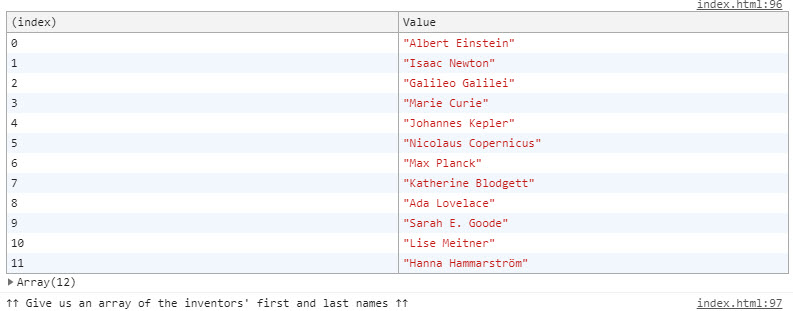
→ forEach()
1 | //要自己產一個陣列,並將值推進去 |
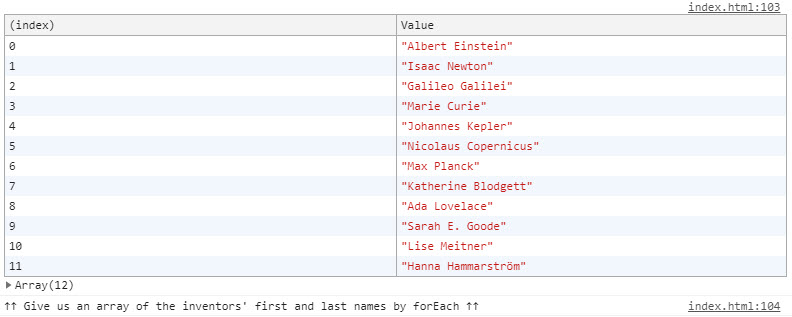
3. 將 inventors 的 birthdate 由大到小排序
→ sort()
語法:arr.sort([compareFunction])
- 若 compareFunction(a, b) 的回傳值小於 0,則會把 a 排在小於 b 之索引的位置,即 a 排在 b 前面。
- 若 compareFunction(a, b) 回傳 0,則 a 與 b 皆不會改變彼此的順序,但會與其他全部的元素比較來排序。
- 若 compareFunction(a, b) 的回傳值大於 0,則會把 b 排在小於 a 之索引的位置,即 b 排在 a 前面。
1 | const ans33 = inventors.sort(function(a, b) { |
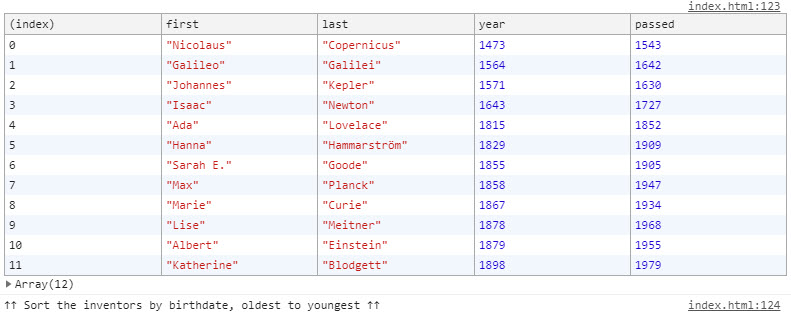
4. 加總所有人的年齡
→ reduce()
1 | const ans4 = inventors.reduce(function(total, inventor) { |

5. 將陣列資料inventors依年齡大小作排序
1 | const ans5 = inventors.sort(function(a, b) { |
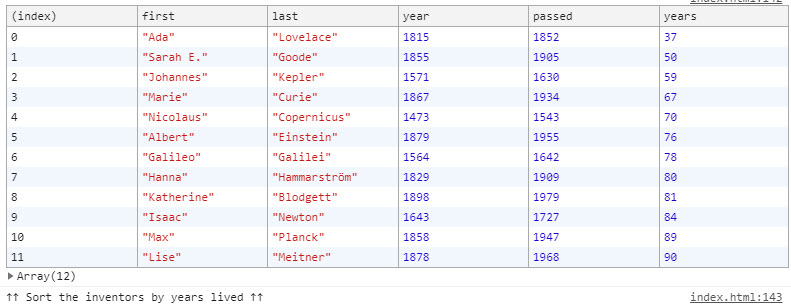
6. 利用 filter(),列出 wiki 資料中所有包含’de’的路名
1 | //先取出路名變為陣列 |
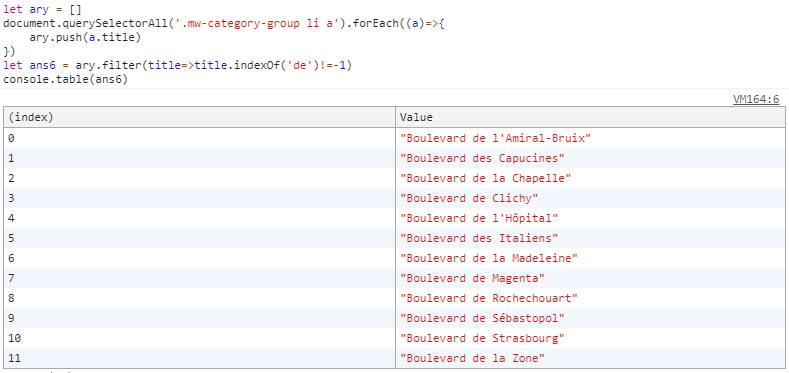
7. 利用 sort(),將 people 依 lastName [字母順序]做排序。
1 | let ans7 = people.sort((a, b) => { |
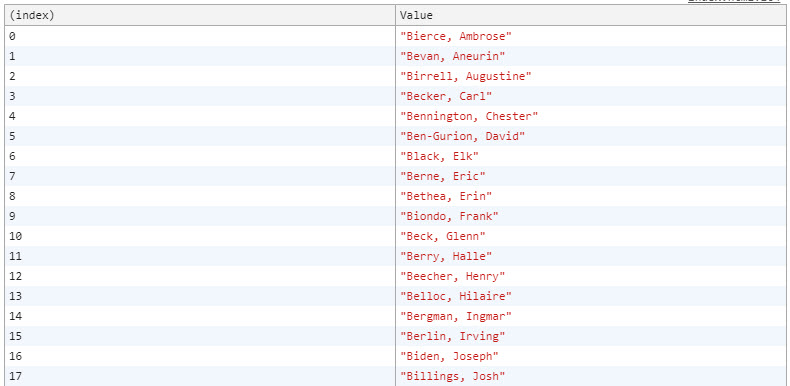
8. 利用 reduce(),計算 data 內每個種類的數量。
1 | const ans8 = data.reduce((obj, content) => { |
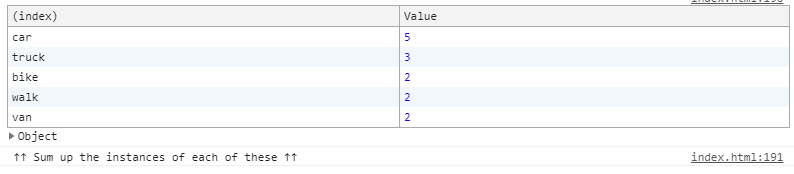
總結
- filter() ==> 回傳一個陣列
- map() ==> 回傳一個陣列
- sort() ==> 回傳一個陣列
- reduce() ==> 回傳一個值